Mathematical Algorithms: From Euclidean to Randomized
- Samvar Shah
- Oct 9, 2024
- 3 min read
Updated: Nov 13, 2024
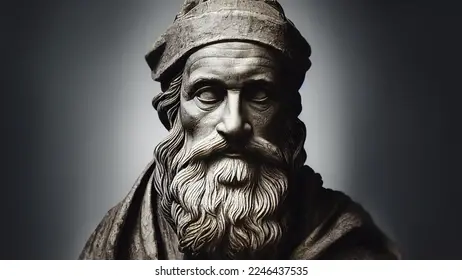
In this post, we'll dive into some foundational and intriguing mathematical algorithms, including the Euclidean Algorithm, Randomized Algorithms, and more.
The Euclidean Algorithm
What It Is: The Euclidean Algorithm is a classic method for finding the greatest common divisor (GCD) of two integers. It is named after the ancient Greek mathematician Euclid, who first described it in his work "Elements."
How It Works: The algorithm is based on the principle that the GCD of two numbers also divides their difference. The process involves repeated division and taking remainders until the remainder is zero. At that point, the non-zero remainder from the previous step is the GCD.
Steps:
Given two numbers a and b where a > b, divide a by b and find the remainder r.
Replace a with b and b with r.
Repeat the process until r becomes zero. The non-zero b is the GCD of the original pair.
Example: To find the GCD of 48 and 18:
48 divided by 18 equals 2 with remainder 12
18 divided by 12 equals 1 with remainder 6
12 divided by 6 equals 2 with remainder 0
The GCD is 6.
Applications: The Euclidean Algorithm is used in number theory, cryptography, and even in computer algorithms for simplification tasks.
Randomized Algorithms
What They Are: Randomized algorithms use random numbers to make decisions during their execution, which can lead to faster solutions for certain problems compared to deterministic algorithms. They leverage probabilistic methods to achieve high efficiency and simplicity.
Types:
Las Vegas Algorithms: These algorithms always produce the correct result, but their running time is probabilistic. An example is the Randomized Quick Sort, which, on average, performs faster than its deterministic counterpart.
Monte Carlo Algorithms: These algorithms use randomness to produce an answer that is correct with a certain probability. For instance, Monte Carlo methods are widely used for numerical integration and optimization problems.
Example: The Randomized Quick Sort algorithm involves selecting a random pivot element for partitioning the data. This approach helps to average out the worst-case scenarios of pivot selection, leading to an expected time complexity of O(n log n).
Applications: Randomized algorithms are used in optimization, cryptography, machine learning, and in scenarios where deterministic solutions are either too slow or impractical.
Other Notable Mathematical Algorithms
1. The Sieve of Eratosthenes
What It Is: This ancient algorithm is used to find all prime numbers up to a given limit. It’s highly efficient for generating a list of primes.
How It Works: The algorithm iteratively marks the multiples of each prime number starting from 2. The numbers that remain unmarked are primes.
Steps:
Create a list of numbers from 2 to n.
Starting with the first number (2), mark its multiples.
Move to the next number that is not marked and repeat until the end of the list.
Applications: The Sieve of Eratosthenes is used in cryptography and for problems involving prime numbers.
2. The Fast Fourier Transform (FFT)
What It Is: FFT is an algorithm to compute the Discrete Fourier Transform (DFT) and its inverse efficiently. It transforms a sequence of values into components of different frequencies.
How It Works: The FFT algorithm reduces the computational complexity of the DFT from O(n^2) to O(n log n), where n is the number of points.
Applications: FFT is widely used in signal processing, image analysis, and solving partial differential equations.
3. The Knuth-Morris-Pratt (KMP) Algorithm
What It Is: This is a string-searching algorithm that searches for occurrences of a "word" within a "text" in linear time.
How It Works: The KMP algorithm preprocesses the word to create a partial match table (or "failure function") to avoid redundant comparisons during the search phase.
Applications: KMP is used in text editors, DNA sequence analysis, and any application requiring efficient substring search.
Very imformative post
Thanks for the visualizers- helps people like me who dont like to read much but are visual learners. The posts are also short which is very helpful.